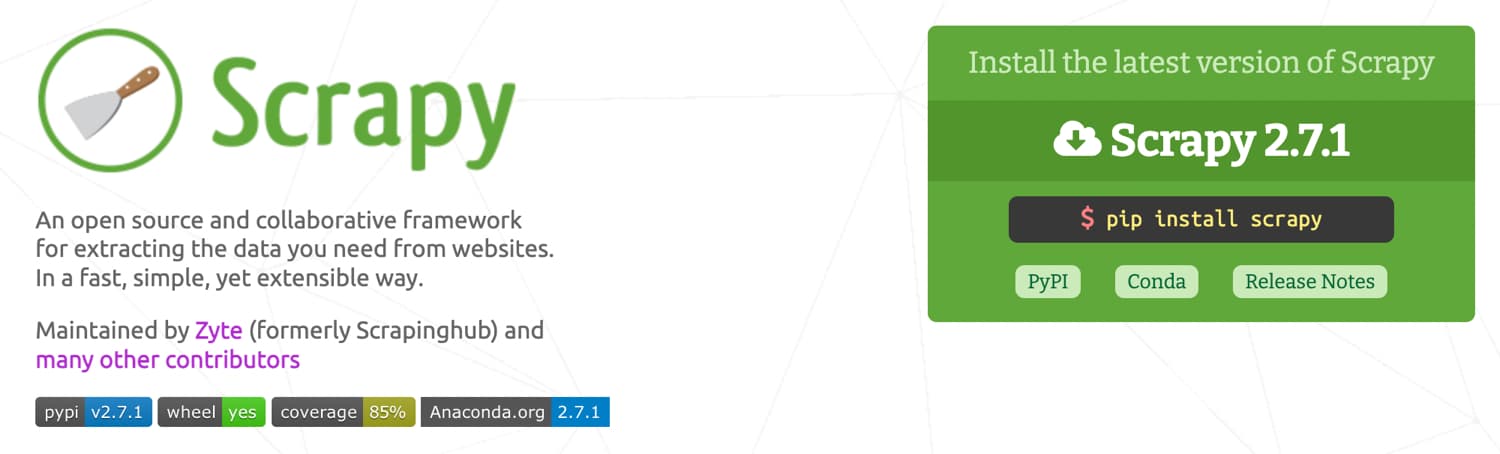
To use Scrapy with a fake user agent, you will need to install the fake-useragent
library and use it to set the user agent in your Scrapy spider. Here's how you can do this:
- Install the fake-useragent library using pip:
pip install fake-useragent
2. In your Scrapy spider, import the fake-useragent
library and use it to generate a fake user agent string:
sdfrom fake_useragent import UserAgent
ua = UserAgent()
fake_user_agent = ua.random
3. Set the USER_AGENT
setting in your Scrapy spider to the fake user agent string:
class MySpider(scrapy.Spider):
name = "myspider"
custom_settings = {
"USER_AGENT": fake_user_agent
}
4. Use the USER_AGENT
setting in your Scrapy spider to set the user agent when making requests:
def start_requests(self):
yield scrapy.Request(
"http://www.example.com",
headers={'User-Agent': self.settings['USER_AGENT']}
)
Why do you need to change user-agent while you are scraping?
There are several reasons why you might want to change the user agent while scraping:
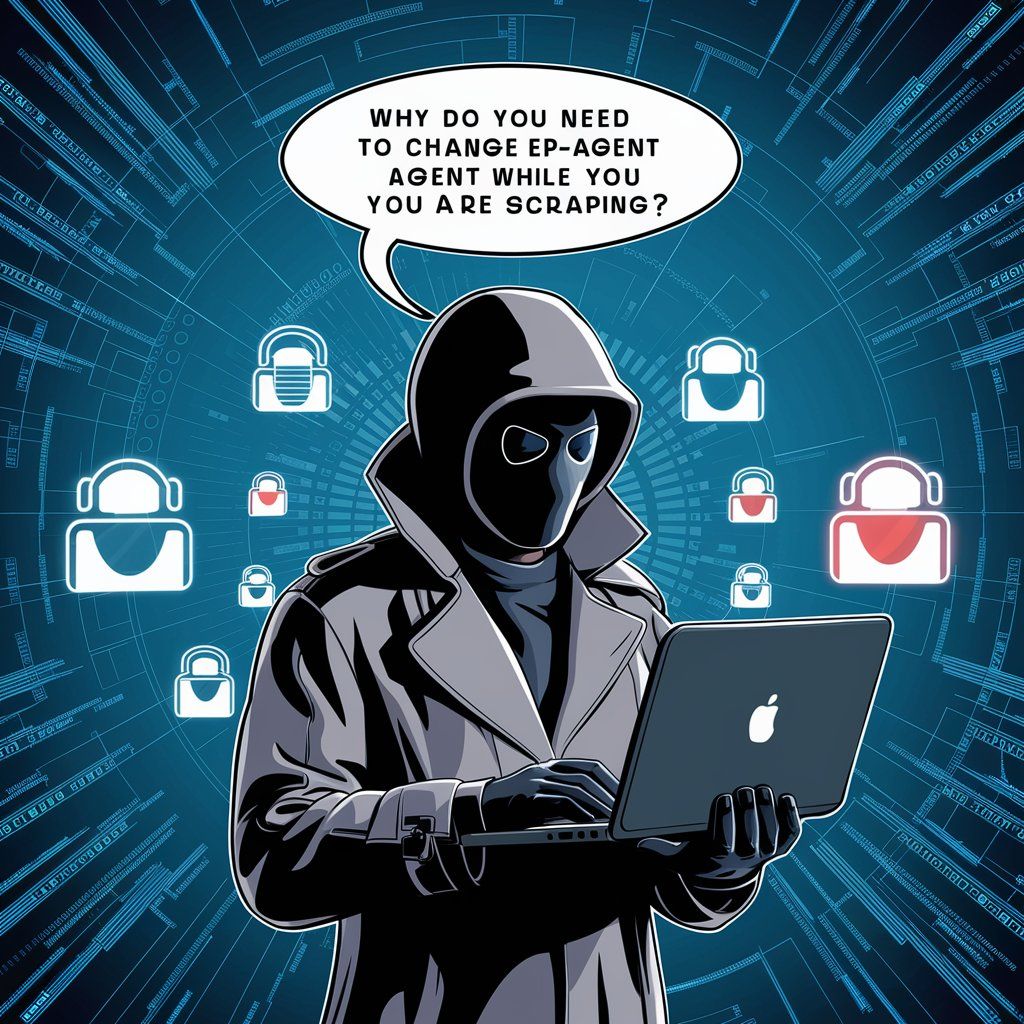
- To avoid being detected as a scraper: Some websites use user agent strings to detect and block scrapers. By changing the user agent, you can make it more difficult for the website to detect that you are a scraper.
- To avoid overloading the website: Some websites may block or rate-limit requests from users with a specific user agent. By changing the user agent, you can avoid being blocked or rate-limited by the website.
- To mimic a specific browser or device: Some websites may serve different content based on the user agent of the request. By changing the user agent, you can mimic a specific browser or device and access content that might not be available to other users.
- To avoid being blocked by anti-scraping tools: Some websites use anti-scraping tools that block requests from specific user agents. By changing the user agent, you can avoid being detected and blocked by these tools.
Overall, changing the user agent can be an effective way to avoid being detected as a scraper and to access content that might not be available to other users. However, it is important to use user agents responsibly and to respect the terms of service and policies of the websites you are scraping.
Using Scrapy with a Fake User Agent
Scrapy, along with a fake user agent, can be a powerful tool to bypass potential roadblocks encountered during web scraping activities. Here are the steps on how to do it:
- Start by installing the fake-useragent library. You can do this using pip, a python package installer.
- Within your Scrapy spider, import the fake-useragent library. This library will be used to generate a synthetic user agent string.
- To assign the fake user agent string, set the USER_AGENT setting within your Scrapy spider accordingly.
- Finally, use the USER_AGENT setting to establish the user agent whenever requests are made by your Scrapy spider.
The Importance of Changing User-Agent
Changing your user agent can greatly aid in carrying out effective web scraping for a variety of reasons:
- Avoiding Detection: Many websites use user agent strings to identify and block web scrapers. Altering the user agent can help mask your scraper and allow it to work undetected.
- Preventing Overload: Some websites might block or limit requests from specific user agents to prevent their servers from being overloaded. Changing your user agent can help avoid such limitations.
- Bypassing Content Restrictions: Certain web content is only available to specific browsers or devices, typically identified by the user agent. When you mimic these using a different user agent, you can access this exclusive content.
- Evading Anti-Scraping Tools: Keeping the user agent variable can help avoid being detected and subsequently blocked by anti-scraping tools used by some websites.
Changing user agent strings can be an effective technique to optimize your web scraping process and circumvent obstacles. However, it's crucial to utilize this responsibly, always respecting the terms of service and privacy policies of the websites you are scraping.
FAQ
What is Scrapy?
Scrapy is an open-source and collaborative web crawling framework for Python. It's primarily used to extract the data from websites and save it to your preferable structure or format.
Why would I use a fake User Agent for web scraping?
Some websites use user agent strings to identify and block web scrapers. Using a fake User Agent can help avoid detection, prevent your scraper from being blocked or limited, mimic specific browsers or devices to access exclusive content, and bypass anti-scraping tools.
How do I install the fake-useragent library?
You can install the fake-useragent library using pip, the Python package installer. Use the command pip install fake-useragent
in your terminal or command line.
Is web scraping illegal?
The legality of web scraping varies depending on the specific circumstances and laws of the country in which it is being carried out. While web scraping public data for legitimate purposes is generally legal, it's important to always respect the site's terms of service and privacy policies. Some websites might prohibit web scraping entirely.
Can I scrape any website?
While technologically possible to scrape any website, not all websites should be scraped. Websites with a robots.txt
file are explicitly asking to not be scraped, and some websites might be protected by copyright laws. It is important to respect these barriers, both for ethical reasons and to avoid potential legal consequences.